概要
Angularでタッチイベントを処理するには、以下をディレクティブに設定することで対応できます。
- (touchstart):タッチしたとき
- (touchmove):タッチしたまま動かしているとき
- (touchend):タッチを話したとき
サンプルコード
ソースコードの概要としては、単純にタッチイベントをリッスンして、なにかしらの処理をするような内容。
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
startY = 0;
moveY = 0;
isEnd = false;
touchStart(event: TouchEvent): void {
this.startY = event.touches[0].clientY;
this.isEnd = false;
}
touchMove(event: TouchEvent): void {
this.moveY = event.touches[0].clientY;
}
touchEnd(event: TouchEvent){
this.isEnd = true;
}
}
<h1
(touchstart)="touchStart($event)"
(touchmove)="touchMove($event)"
(touchend)="touchEnd($event)">
タッチイベントの範囲
</h1>
<p>
startY:{{startY}}
moveY:{{moveY}}
isEnd:{{isEnd}}
</p>
動作
開発者ツールでタッチイベントを発生させると、タッチした個所の座標などを表示できることがわかった。
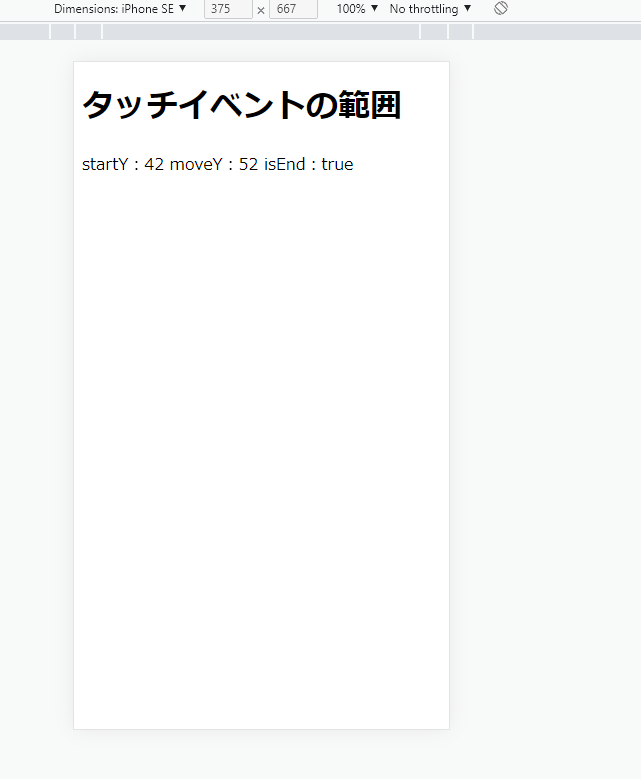