インターセプターについて
HttpClientによるhttp通信の処理で、どのapi呼び出しでも、共通の処理を行いたいといったときに、インターセプターの機能が便利です。
実装
interceptor
custom.intercepter.tsファイルを作成し、CustomInterceptor関数を作成します。
export function CustomInterceptor(req: HttpRequest<unknown>, next: HttpHandlerFn): Observable<HttpEvent<unknown>> {
// リクエストのURLを出力
console.log(req.url);
return next(req).pipe(tap(event => {
if (event.type === HttpEventType.Response) {
// レスポンスのステータスコードを出力
console.log(event.status);
}
}));
}
上記では、リクエスト直前の共通処理、レスポンスの共通処理を書きます。
レスポンスの共通処理は、pipeの中に書きます。
withInterceptorsでprovidersに加える
app.config.tsのprovidersに、provideHttpClientを設定し、その引数にwithInterceptorsを使って、先ほど作ったインターセプターを設定します。
export const appConfig: ApplicationConfig = {
providers: [
provideZoneChangeDetection({ eventCoalescing: true }), provideRouter(routes),
provideHttpClient(withInterceptors([CustomInterceptor]))
]
};
APIコールの実行処理
以下のように、httpClientで、getメソッドを呼んで、subscribeすると、
インターセプターの共通処理が出力されます。
export class AppComponent implements OnInit {
constructor(private httpClient: HttpClient){
}
ngOnInit(): void {
this.httpClient.get("https://{url})
.subscribe((res) => console.log(res))
}
}
コンソール出力を見ると、インターセプターで設定したURLやステータスコードの出力が行われていることが確認できました。
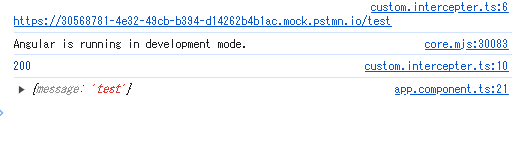