作ったテーブル
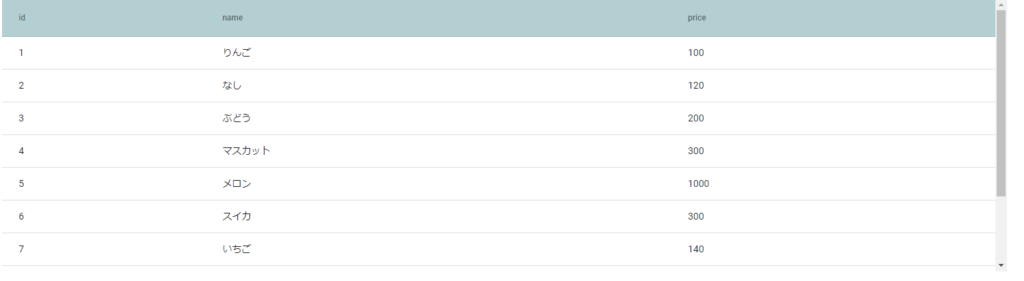
ソースコード
app.module.ts
ここでは、MatTableModuleをインポートする。
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { MatTableModule } from '@angular/material/table'; // 追加
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule,
MatTableModule // 追加
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
app.compnent.ts
テストデータを連想配列で作成する。
import { Component } from '@angular/core';
export interface FoodModel {
name: string;
id: number;
price: number;
}
const FOOD_DATA: FoodModel[] = [
{id: 1, name: 'りんご', price: 100},
{id: 2, name: 'なし', price: 120},
{id: 3, name: 'ぶどう', price: 200},
{id: 4, name: 'マスカット', price: 300},
{id: 5, name: 'メロン', price: 1000},
{id: 6, name: 'スイカ', price: 300},
{id: 7, name: 'いちご', price: 140},
{id: 8, name: 'もも', price: 150},
{id: 9, name: 'バナナ', price: 18},
{id: 10, name: 'ブルーベリー', price: 200},
];
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
columnName = ['id', 'name', 'price'];
dataSource = FOOD_DATA;
}
app.component.html
ここでテンプレートの設定をしています。
<section class="container">
<table mat-table [dataSource]="dataSource">
<ng-container matColumnDef="id">
<th mat-header-cell *matHeaderCellDef> id </th>
<td mat-cell *matCellDef="let data"> {{data.id}} </td>
</ng-container>
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef> name </th>
<td mat-cell *matCellDef="let data"> {{data.name}} </td>
</ng-container>
<ng-container matColumnDef="price">
<th mat-header-cell *matHeaderCellDef> price </th>
<td mat-cell *matCellDef="let data"> {{data.price}} </td>
</ng-container>
<tr class="header-row" mat-header-row *matHeaderRowDef="columnName; sticky: true"></tr>
<tr mat-row *matRowDef="let row; columns: columnName;"></tr>
</table>
</section>
- [dataSource]に連想配列のデータを入れると、そのデータを1レコードずつ表示します。
- ng-containerでセルの設定する値を入れます。
- 最後にtr属性にmat-header-rowでヘッダー行の設定、mat-rowで通常の行の設定を加えられます。
app.component.scss
スタイルで、色やスクロールなどの調整をしています。
.container {
height: 400px;
width: 80%;
overflow: auto;
margin: auto;
}
table {
width: 100%;
}
.header-row {
background-color: rgb(179, 207, 209);
}