はじめに
Angular Materialには、MatSelectというセレクトボックスがあります。
そのセレクトボックスの項目リストに対して、検索キーワードを入れて、項目リストをフィルタする機能を作っていこうと思います。
実装方法
環境
- Angular18
コンポーネントの作成
import { NgFor } from '@angular/common';
import { Component, ElementRef, ViewChild } from '@angular/core';
import { FormControl, FormsModule, ReactiveFormsModule } from '@angular/forms';
import { MatIconAnchor, MatIconButton } from '@angular/material/button';
import { MatFormFieldModule } from '@angular/material/form-field';
import { MatInputModule } from '@angular/material/input';
import { MatSelectModule } from '@angular/material/select';
import { MatIconModule } from '@angular/material/icon';
@Component({
selector: 'app-filter-select',
standalone: true,
imports: [
ReactiveFormsModule,
FormsModule,
MatSelectModule,
MatFormFieldModule,
MatInputModule,
MatIconModule,
NgFor
],
templateUrl: './filter-select.component.html',
styleUrl: './filter-select.component.scss'
})
export class FilterSelectComponent {
items: string[] = ['Apple', 'Orange', 'Banana', 'Grape', 'Pineapple'];
filteredItems: string[] = [...this.items];
filterValue: string = '';
selectedItem: string | null = null;
applyFilter() {
this.filteredItems = this.items.filter(item =>
item.toLowerCase().includes(this.filterValue.toLowerCase())
);
}
}
フィールドやメソッドの概要については以下の通りです。
- items:リスト項目
- filteredItems:フィルタされたリスト項目
- filterValue:検索キーワード
- selectedItem:選択している項目
- applyFilter():入力イベントを受け取って、リストをフィルタする
テンプレートの作成
<mat-form-field>
<mat-label>選択してください</mat-label>
<mat-select [(value)]="selectedItem">
<mat-icon class="search-icon" matSuffix>search</mat-icon>
<input
class="filter"
matInput
[(ngModel)]="filterValue"
(ngModelChange)="applyFilter()"
/>
<mat-option *ngFor="let item of filteredItems" [value]="item">{{ item }}</mat-option>
</mat-select>
</mat-form-field>
<p>選択したアイテム:{{selectedItem}}</p>
やり方としては、セレクトボックスの中に、inputの入力エリアを作り、そこのテキスト入力値で、フィルターをかけています。
scss
.mat-mdc-form-field-input-control.mat-mdc-form-field-input-control.filter {
border-width: 0px 0px 5px 0px;
border-color: black;
border-style: solid;
padding-left: 24px;
position: sticky;
background-color: #efedf0;
top: 0;
z-index: 1;
}
.search-icon {
position: absolute;
z-index: 2;
}
配置やデザインを調整しつつ、リストをスクロールしても、検索の入力エリアはスクロールされないように修正しています。
実行結果
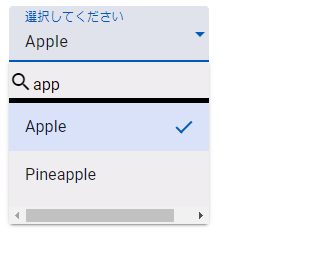
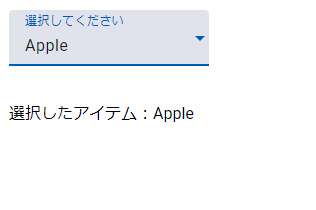
まとめ
このような形で、セレクトボックスの項目リストに対してフィルタをかけることができました。
あとは、自分の好みに合わせてデザインなどを調整していけばよいと思います。